YouTube to MP3 Converter: A Comprehensive Guide
Looking for a reliable YouTube to MP3 converter? Learn how to effortlessly convert YouTube videos to MP3 format for free with our step-by-step guide.
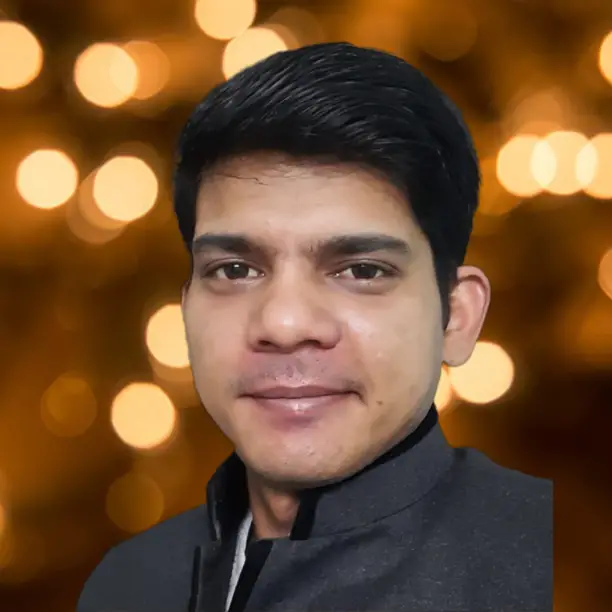
ย
In JavaScript, we use arrays to manage and manipulate collections of data. Whether youโre experienced or new to coding, knowing how to check if array is empty javascript is important for developers.
One common task every JavaScript developer encounters is the need to check whether an array is empty. In this manual, weโll explore various methods to effectively accomplish this task. This will make sure your JavaScript code works well and is fast.
Ensure the array is not vacant before utilizing it to avert issues or unforeseen conduct when initiating a task. Picture yourself developing a dynamic web application that relies heavily on user input and data manipulation.
Before using arrays, check if array is empty javascript prevents errors and enhances user experience. This will make the code run better and the application more reliable. You can check if an array is empty by looking at its length.
One of the most straightforward methods to check if an array is empty is by leveraging the โlengthโ property. In JavaScript, the โlengthโ property returns the number of elements in an array. By comparing the โlengthโย to zero, we can easily determine whether the array is empty or not.
ย
const myArray = []; if (myArray.length === 0) { ย ย console.log("The array is empty."); } else { ย ย console.log("The array is not empty."); }
ย
To ensure robustness in our code, we can combine the โisArrayโ method with the โlengthโย property. This method checks if the variable is an array and if its length is zero, adding an extra validation step.
const myArray = []; if (Array.isArray(myArray) && myArray.length === 0) { ย ย console.log("The array is empty."); } else { ย ย console.log("The array is not empty."); }
ย
Also Learn:
ย
The โeveryโย method is another powerful tool in our arsenal for array manipulation. It tests whether all elements in the array pass the provided function. When the array is empty, the โeveryโย method returns โtrue, โ indicating no elements to evaluate.
ย
const myArray = []; if (myArray.every(element => !element)) { ย ย console.log("The array is empty."); } else { ย ย console.log("The array is not empty."); }
ย
Conversely, the โsomeโ method offers an alternative approach to checking for an empty array. The โsomeโ method checks if any element in an array meets a condition. It returns โfalseโย if the array is empty.
ย
const myArray = []; if (!myArray.some(element => element)) { ย ย console.log("The array is empty."); } else { ย ย console.log("The array is not empty."); }
ย
In JavaScript programming, ensuring an array is not empty is crucial for maintaining code reliability and preventing errors. Conducting a JavaScript check if array is empty is important for defensive programming, helping developers validate data states efficiently. Developers can prevent issues and ensure smooth JavaScript code by using techniques to check if an array is empty.
In short, JavaScript developers need to know how to quickly check if array is empty javascript. This skill is valuable for developers of all levels. By using these methods in your code, you can make sure your JavaScript apps are strong, bug-free, and run efficiently. Whether youโre making basic scripts or advanced web apps, learning these techniques will improve your JavaScript skills significantly.
Remember, the key to writing exceptional JavaScript code lies in prioritizing readability, efficiency, and maintainability. Thus, continue to test, discover, and refine your abilities. Enjoy the coding journey!
Follow Us on LinkedInย for the Latest Updates.
Got questions? Ask them here and get helpful answers from our community!