Learn C Programming
Unions
As a new programmer, you may have come across โunionโ in C.ย In this tutorial, we will explore what are c unions, how they work, and why they are useful. Whether youโre a student, a teacher, or a beginner in programming, this guide will provide you with a comprehensive understanding of unions in C programming.
Introduction
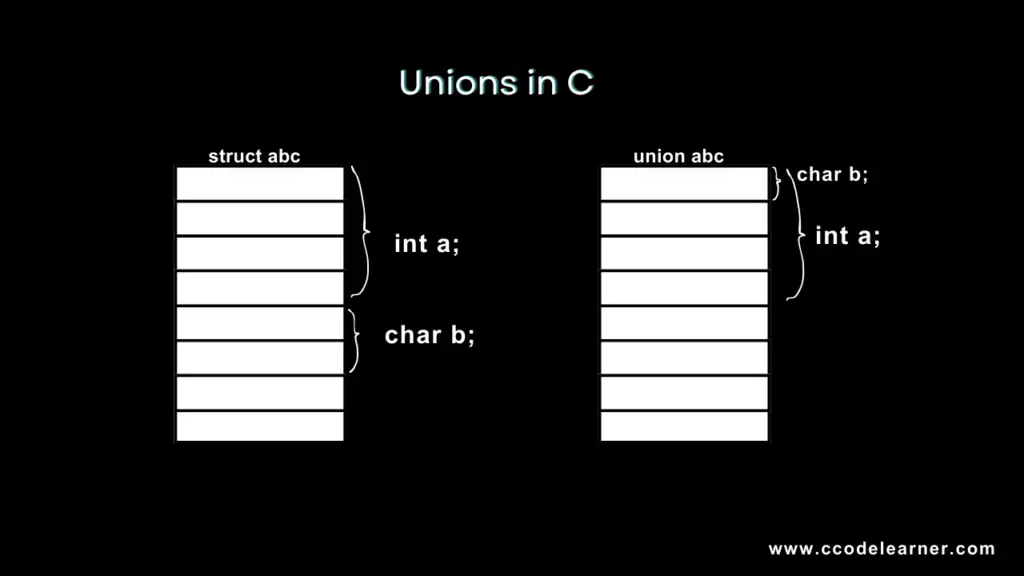
C Unions, like structures, are user-defined data types in C. They allow you to combine different data types into a single variable, but with a crucial difference โ while structures allocate memory for each member variable, unions share memory among its members. This means that a union variable uses enough memory to hold its largest member.
The purpose of this tutorial is to delve deeper into the concept of unions and explore various aspects of their usage. We will discuss the syntax, memory allocation, access patterns, and potential pitfalls when working with unions. By the end of this tutorial, you will have a solid understanding of unions and how to use them effectively in your C programs.
The Syntax of C Unions
To define a union in C, you use the union
keyword followed by the unionโs name, just like when defining a structure. Inside the union, you list the member variables, also known as fields, each with its own data type. Letโs take a look at an example:
union MyUnion {
int myInt;
float myFloat;
char myChar;
};
In the above example, we define a union called โMyUnionโ with three member variables: an integer (myInt
), a float (myFloat
), and a character (myChar
).
Memory Allocation in C Unions
As mentioned earlier, unions allocate memory based on the size of their largest member. This means that the memory allocated for a union can be accessed using any of its member variables, but only one member can be accessed at a time. When you modify a member variable, you may overwrite the contents of other member variables.
To understand this better, letโs consider the following example:
union MyUnion {
int myInt;
float myFloat;
char myChar;
};
union MyUnion u;
u.myInt = 42;
printf("Value of myInt: %d\n", u.myInt);
u.myFloat = 3.14;
printf("Value of myFloat: %f\n", u.myFloat);
printf("Value of myInt after modifying myFloat: %d\n", u.myInt);
In this example, we declare a union called โMyUnionโ and create a variable of that type called u
. We assign a value of 42 to myInt
and print it out. Then, we assign 3.14 to myFloat
and print it out. Finally, we print the value of myInt
again and observe that it has changed. This is because modifying myFloat
overwrites the memory used by myInt
.
Accessing Union Members
You can access the members of a union using the dot operator (.
) just like you would with a structure. However, you need to be careful when accessing union members, as undefined behavior can occur if you read from one member and write to another without changing the type properly. Letโs see an example:
union MyUnion {
int myInt;
float myFloat;
};
union MyUnion u;
u.myInt = 42;
printf("Value of myInt: %d\n", u.myInt);
u.myFloat = 3.14;
printf("Value of myFloat: %f\n", u.myFloat);
printf("Value of myInt after modifying myFloat: %d\n", u.myInt);
In this example, we define a union called โMyUnionโ with two members โ an integer and a float. We assign a value to myInt
and print it out. Then, we assign a value to myFloat
and print it out as well. However, notice that we are trying to print the value of myInt
again after modifying myFloat
. This results in undefined behavior because the memory that myFloat
occupies is different from the memory that myInt
was using.
To avoid undefined behavior, itโs important to only access the member that was most recently modified. If you need to access a different member, you should reassign a value to it first. For example:
union MyUnion {
int myInt;
float myFloat;
};
union MyUnion u;
u.myInt = 42;
printf("Value of myInt: %d\n", u.myInt);
u.myFloat = 3.14;
printf("Value of myFloat: %f\n", u.myFloat);
u.myInt = 69; // Assign a new value to myInt
printf("Value of myInt after modifying myFloat: %d\n", u.myInt);
In this revised example, we assign 69 to myInt
after modifying myFloat
. Now, when we print the value of myInt
, we see the correct value. Itโs crucial to remember this rule when working with unions to avoid unexpected results and bugs in your code.
Also Learn:
Practical Use Cases of C Unions
Now that you understand unions and how they work, letโs explore some practical use cases where unions can be useful.
1. Storing Multiple Types of Data
Unions can be used to store different types of data in a compact manner. For example, in a messaging application, you may want to represent a message as either text or an image. By defining a union with member variables for both text and image data, you can store either type of data in a single variable.
union MessageData {
char text[256];
struct {
unsigned char* imageData;
unsigned int width;
unsigned int height;
} image;
};
In the above example, we define a union called โMessageDataโ with two member variables. The text
member is an array of characters that can hold up to 255 characters, while the image
member is a nested structure that represents image data. By using this union, you can store either text or image data in a single variable, depending on the context.
2. Efficient Memory Usage
Unions can also be used to optimize memory usage when dealing with different data types. For instance, if you have a data structure that can be either an integer or a floating-point number, you can define a union to save memory by sharing the same memory space for both types.
union Number {
int intValue;
float floatValue;
};
In this example, the union โNumberโ allows you to store either an integer or a float value in the same memory. This can be beneficial when memory optimization is a concern, especially in embedded systems or applications with limited resources.
3. Bit Manipulation
Unions can be helpful when working with individual bits or specific fields within a variable. By defining a union with members of different sizes, you can manipulate bits at a granular level, which can be useful in scenarios such as compression algorithms, network protocols, or low-level hardware interaction.
union Flags {
struct {
unsigned int flagA : 1;
unsigned int flagB : 1;
unsigned int flagC : 1;
unsigned int reserved : 29;
} bits;
unsigned int rawValue;
};
In this example, we define a union called โFlagsโ with two members โ bits
and rawValue
. The bits
member is a nested structure that represents the individual flags, each represented by one bit, along with a reserved field. The rawValue
member is an unsigned integer that provides direct access to the binary representation of the flags. This union can be used to manipulate the individual flags easily, providing efficient control over specific bits within a larger data structure.
Conclusion
In this tutorial, we explored unions in C programming. We covered the syntax of c unions, memory allocation, accessing union members, and their practical use cases. Unions are a powerful tool that allows you to combine different data types into a single variable, optimizing memory usage and enabling efficient bit manipulation.
Remember to use c unions carefully, as accessing members incorrectly can lead to undefined behavior. In c unions, always assign values before accessing different members and be cautious of their memory-sharing nature.
With C unions, you can optimize memory usage and manipulate individual bits, making them a valuable addition to your programming toolbox. Use this feature in your projects for applications and memory optimization.
Happy coding, and may your unions always share the right memory!
Follow Us onย LinkedInย for the Latest Updates.
ย